Have you ever wondered how you could set up multiple queues using the same connection? Let's figure out why and how.
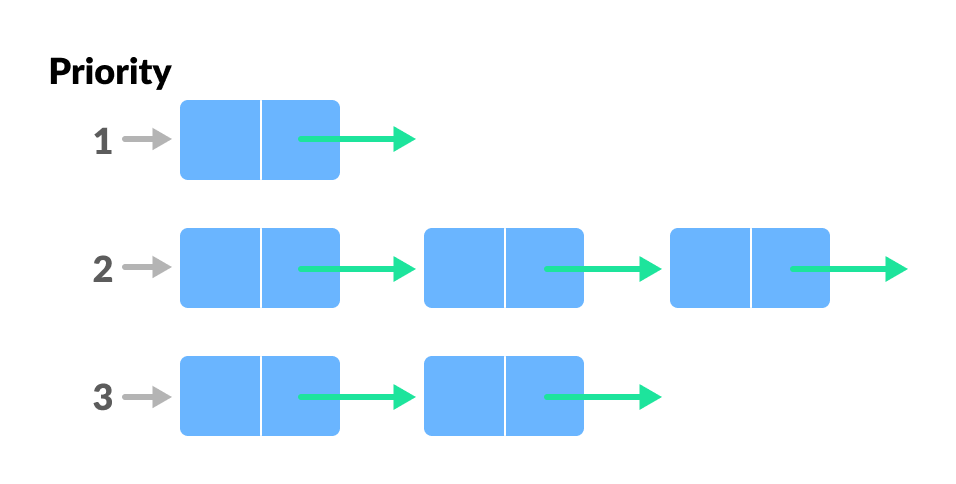
Why Use Multiple Connections for Laravel Queues?
Sometimes your application needs to process different jobs with a higher priority than others. To do this, Laravel enables you to specify queue names and give them priorities as you wish.
Imagine you have jobs like SendVerificationCode
, BroadcastWeeklyNewsletter
and ProcessPayment
. You obviously don't want jobs that process payments to wait until newsletter jobs are complete.
How to Define Multiple Queues to Prioritize Jobs
First, you should know which connection you intend to use for all of these queues. Personally I am more acustomed to database
or Amazon sqs
connections:
// config/queue.php
'database' => [
'driver' => 'database',
'table' => 'jobs',
'queue' => 'default',
'retry_after' => 90,
],
And I will obviously define in the .env
file that I will be using the database connection.
Now, whenever we dispatch any of the jobs, they will consume the default
queue as indicated in the configuration above.
But here is the order of priority we want our imaginary jobs to be processed:
- Highest priority - ProcessPayment
- Medium priority - SendVerificationCode
- Low (or default) priority - BroadcastWeeklyNewsletter
Then when we dispatch the jobs, we will define the queue names explicitly
use App\Jobs\{
BroadcastWeeklyNewsletter,
ProcessPayment,
SendVerificationCode,
};
// This job is sent to the connection's default queue...
BroadcastWeeklyNewsletter::dispatch();
// This job is sent to the connection's "payments" queue...
ProcessPayment::dispatch()->onQueue('payments');
// This job is sent to the connection's "verification" queue...
SendVerificationCode::dispatch()->onQueue('verification');
Lastly we need to tell the workers the order of priority from first to last:
php artisan queue:listen database --queue=payments,verification,default
That's all. Now all your queue workers will process all payment jobs first before all the other jobs.
Similarly, all code verification jobs will be processed ahead of the newsletter jobs.
Join to participate in the discussion